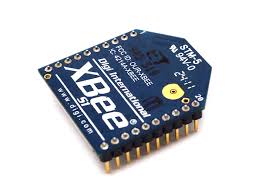
How to connect XBee S1 to Arduino Nano (API mode)
Objective
To develop an API mode application of XBee S1 module using Arduino Uno/Nano/Pro Mini.
Prerequisite
This tutorial assumes that you are familiar with the basic operation of XBee S1 module. Please visit basic tutorial on XBee S1 if you are not.
Description of Project
1. Transmitter transmits a message periodically, which contains data or information to be delivered to the receiver.
2. Receiver extracts this data or information from the delivered message.
3. Transmitter is powered by battery only and it stays in SLEEP mode to save electric energy when it does not transmits a message.
4. Receiver is not powered by battery and always stays awake.
Test Environment
- PC with MS Windows 7 Professional K (64-bit)
- CodeVisionAVR or Atmel Studio 6
- TeraTerm or other terminal program
- X-CTU
Step I: To Understand API mode operation of XBee S1
- The XBee S1 goes to sleep mode if the SM parameter is 1 or 2 and voltage at pin 9 is VCC. By default, the voltage at pin 9 is VCC if this pin is not connected.
- The XBee S1 wakes up from sleeping mode by applying GND level voltage to pin 9 and stays in awake mode as long as voltage at pin 9 is GND level.
- The SM parameter value can be changed to 0 while voltage at pin 9 is GND level.
1. Connect one Xbee S1 module (transmitter) to a PC through FT232 module as shown below.
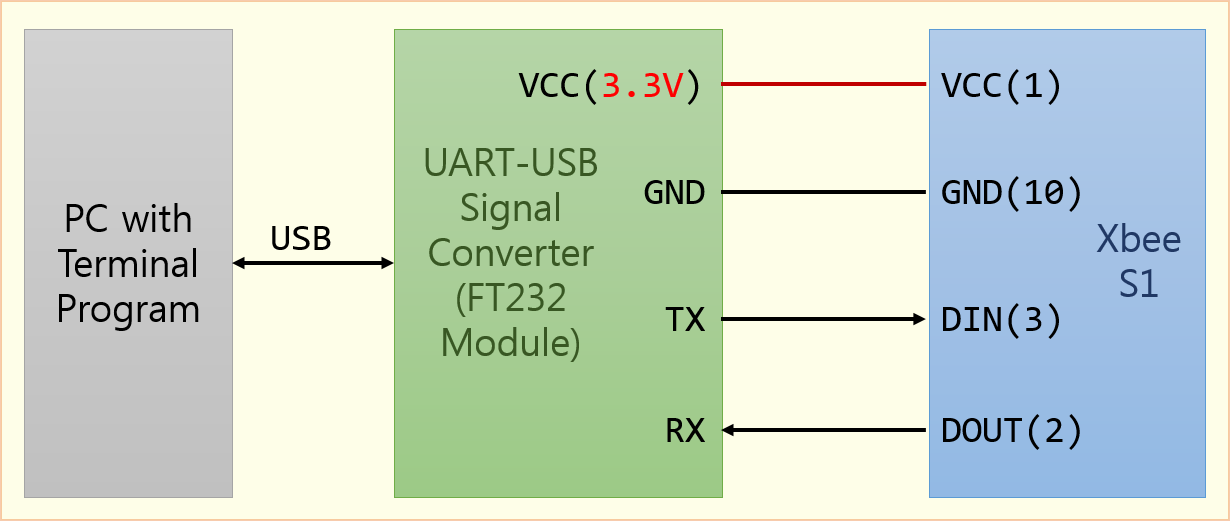
2. Configure the XBee S1 module (transmitter) as following.
A. Run X-CTU program.
B. Click "Add Device" button at the upper left corner of the X-CTU window.
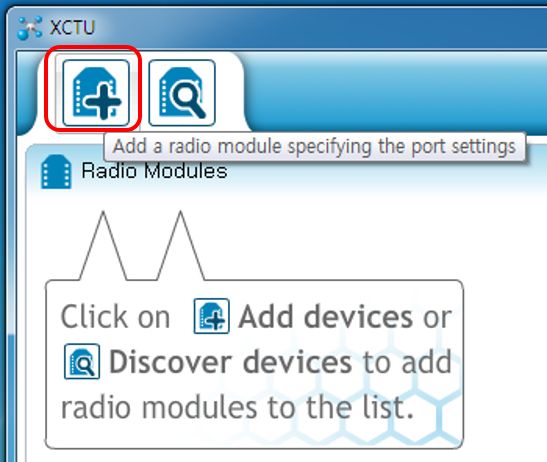
C. Select the virtual COM port number, Baud Rate, Data Bits, Parity, Stop Bits, Flow Control as indicated below and click "Finish" button. The virtual COM port number may be different depending on your system.
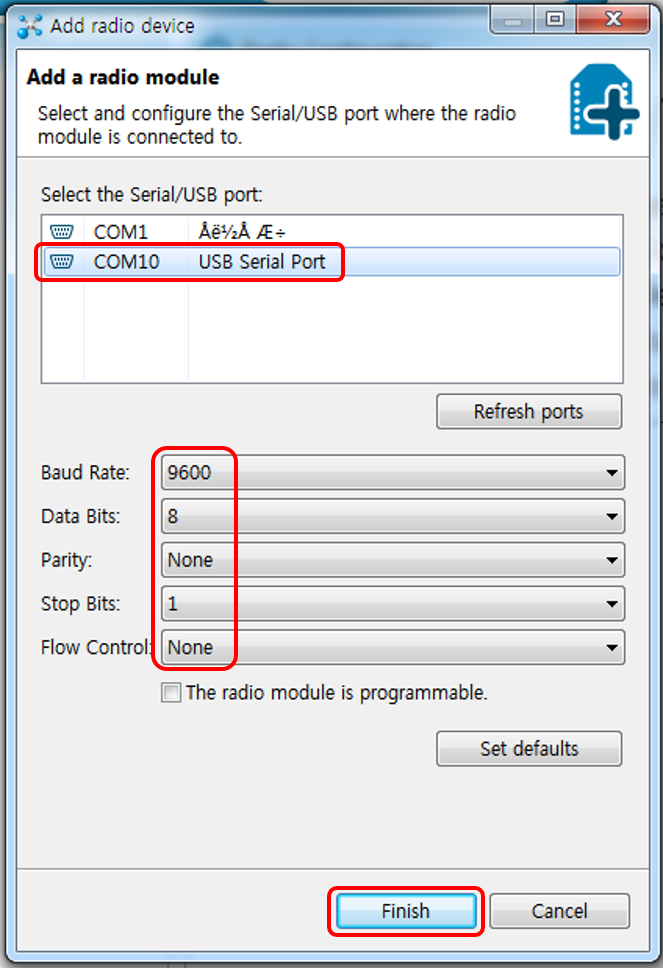
D. X-CTU shows information regarding the detected XBee S1 module after scanning. Click this information box pointed by the red color arrow as shown below.
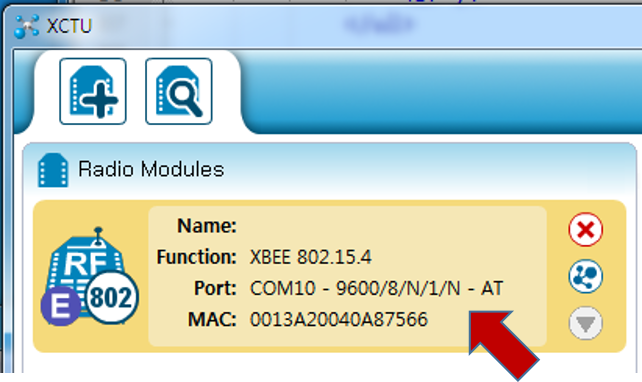
If X-CTU cannot detect any XBee S1 module, it is considered that the default setting values of the module have been changed.
(1) If the default communication parameters have been changed, repeat step C with different communication parameters, for example, different "Baud Rate".
(2) If SM parameter has been changed from its default value (0x00, non-sleeping mode) to different value, for example, 0x01 or 0x02 (both of them are for sleeping mode), connect pin 9 to GND and repeat step (3).
E. Radio Configuration panel will be appeared on the right pane.
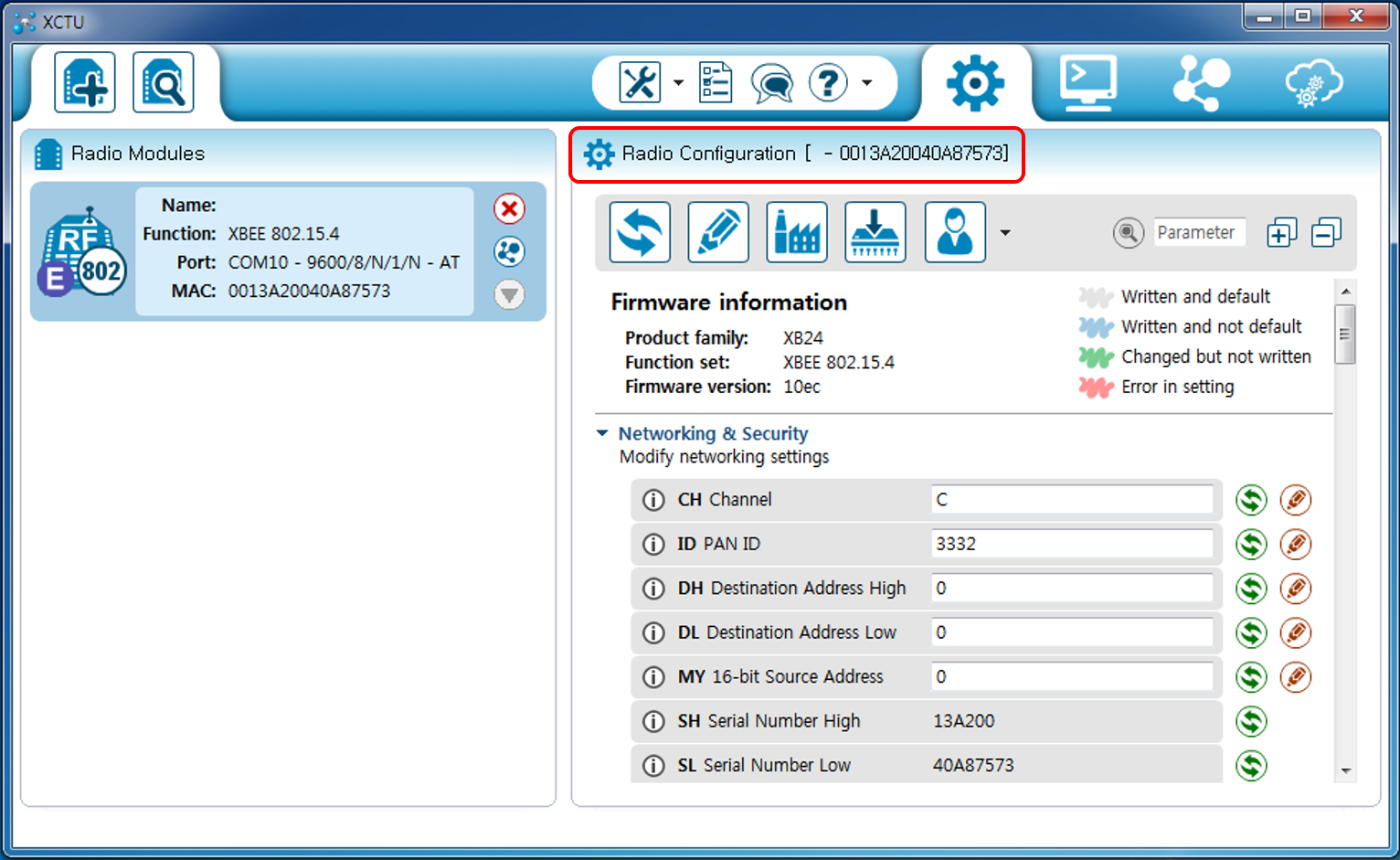
F. Change MY 16-bit Source Address to 1234 (in hexadecimal).
This 16-bit value will be used as a unique address of this device (transmitter).
Take a note of this address for the future application.
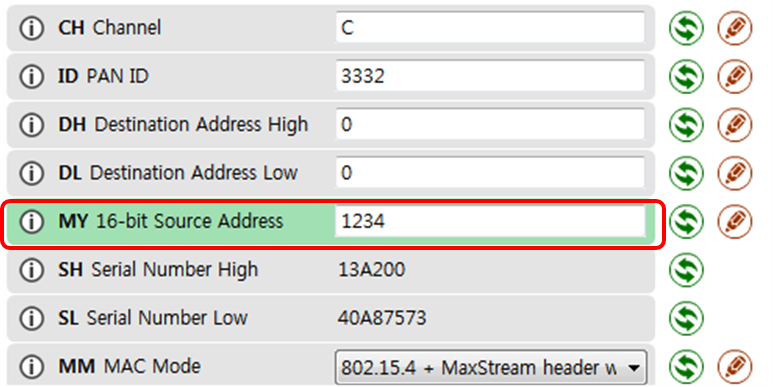
G. Take a note of SH and SL values (in hexadecimal) for the future application.
This 32-bit value will be used as another unique address of this device (transmitter) when the 16-bit address (MY value) is not used.
In this example, SH is 0x0013A200 and SL is 0x40A87573.
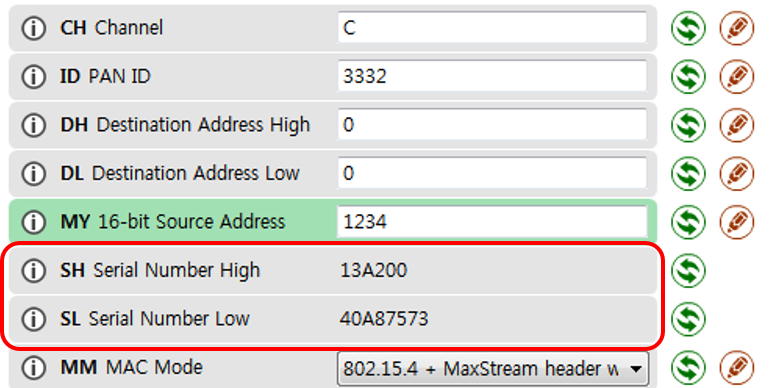
H. Change AP to API enabled [1].
This setting will let this XBee S1 module operate in API mode (without escaped characters).
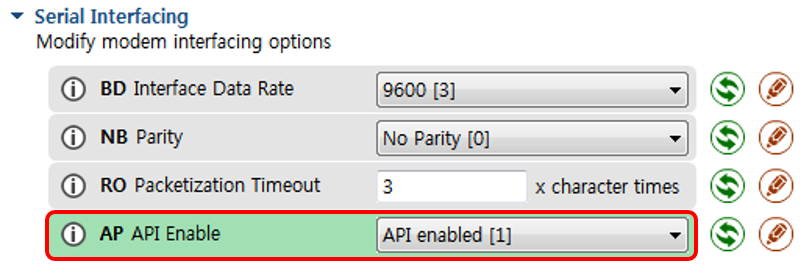
I. Click Write radio settings icon at the top to write new setting values to the non-volatile memory.
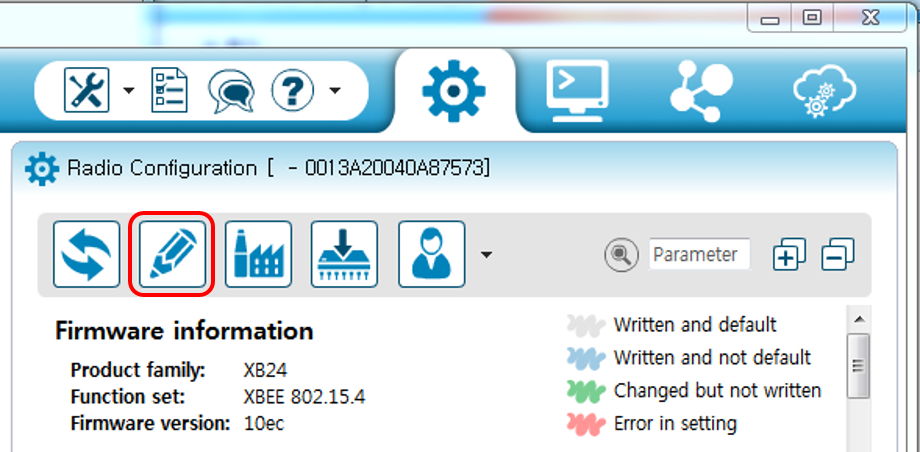
3. Switch to console mode by clicking Switch Consoles Working mode icon.
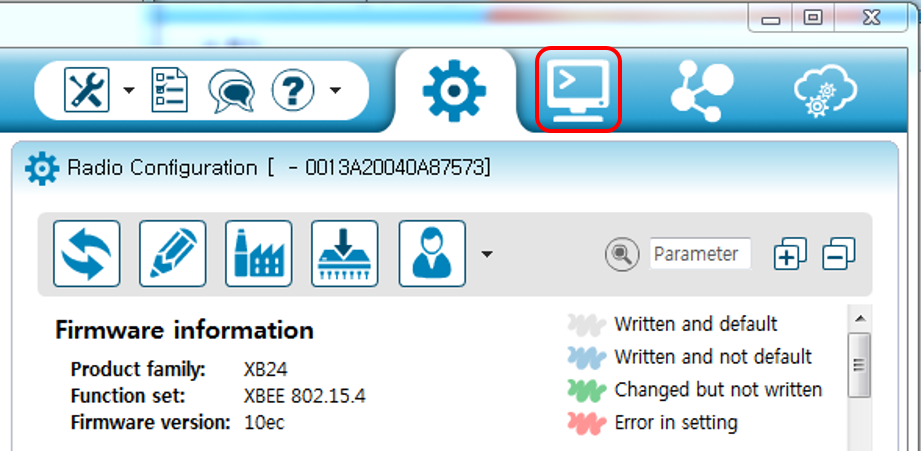
4. Click the Open the serial connection with the radio module icon to connect the XBee S1 module to the console.
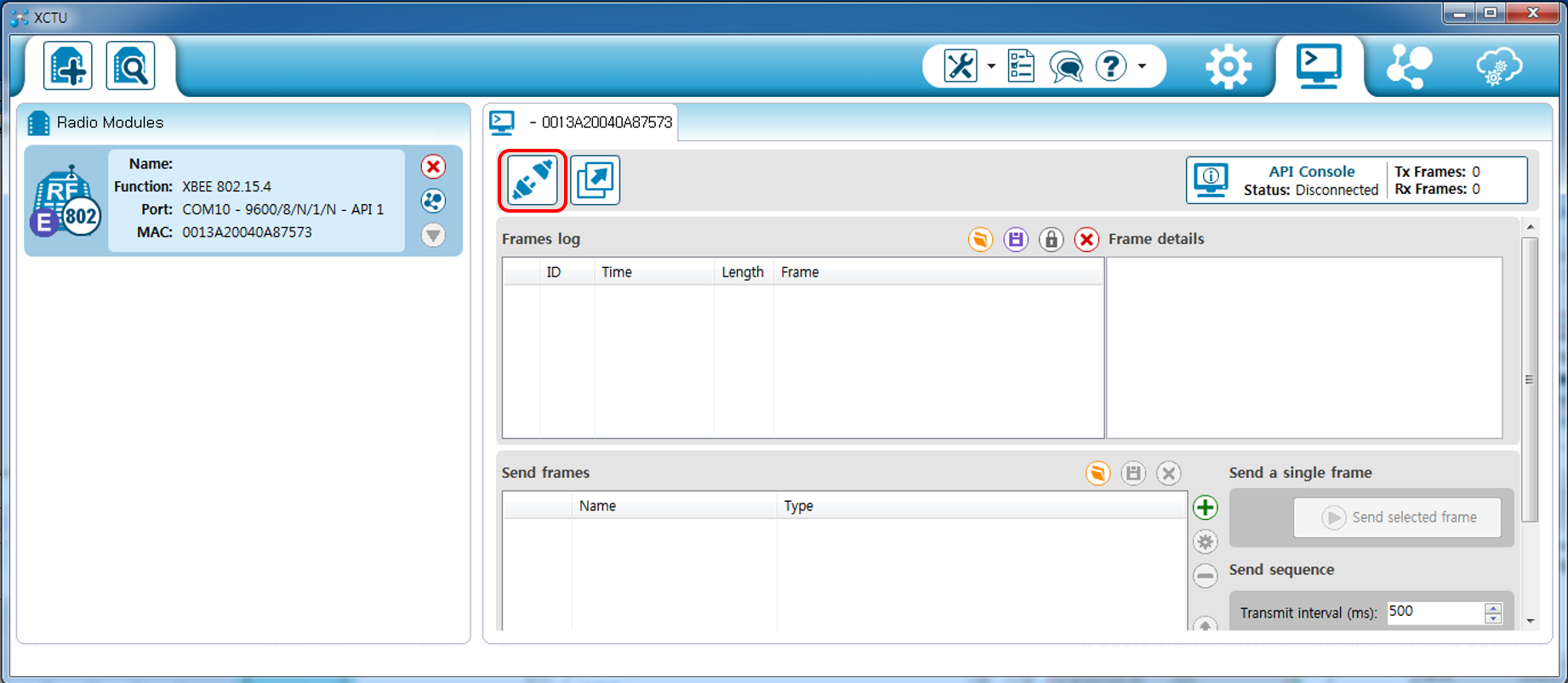
5. You can find the connection icon shape changed and also the status changed to Connected.
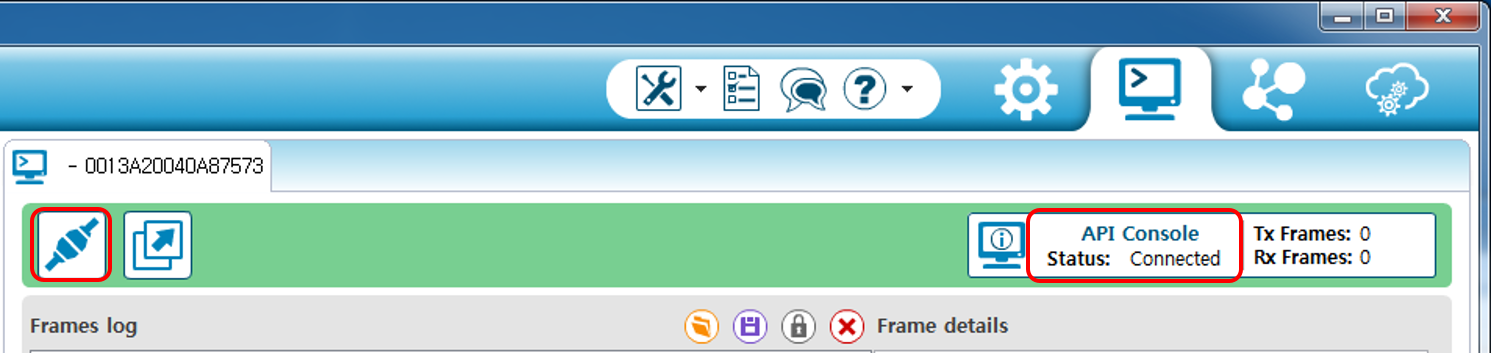
6. Click Add new frame to the list button to create a new message frame.
(It is marked with a green color + symbol with a green circle.
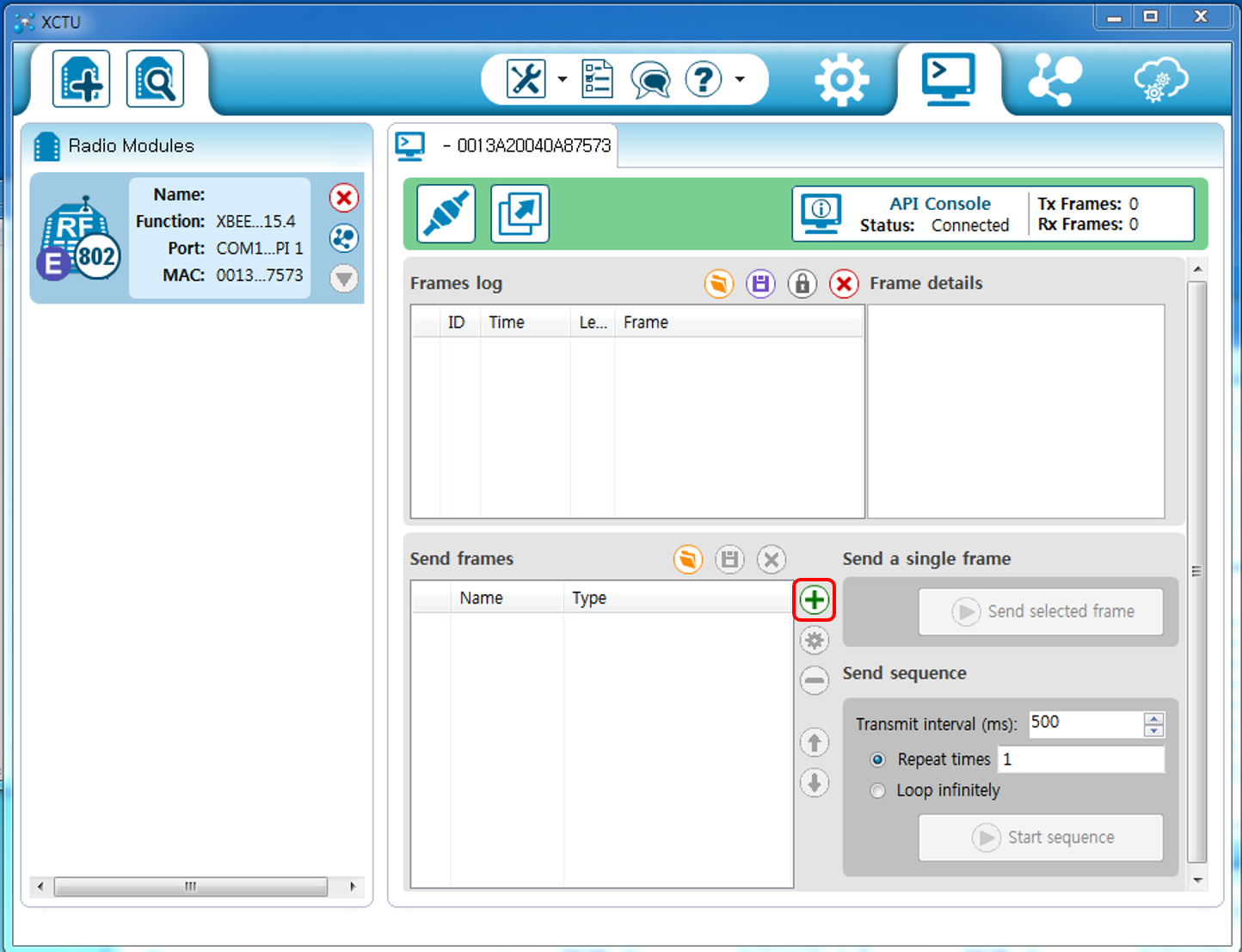
7. Add API frame to the list dialog will be popped up. Click Create frame using 'Frame Generator' tool... button to open wizard.
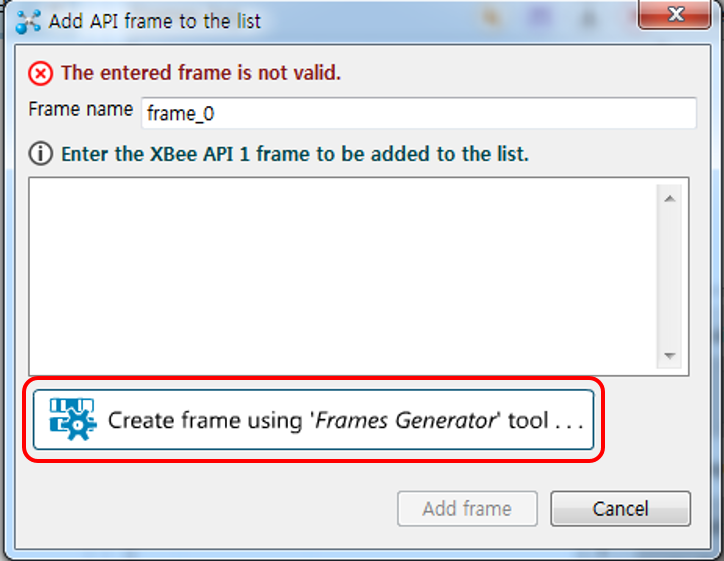
8. XBee API Frame Generator dialog will be popped up.
Click Frame type and select 0x01 - TX (Transmit) Request: 16-bit address.
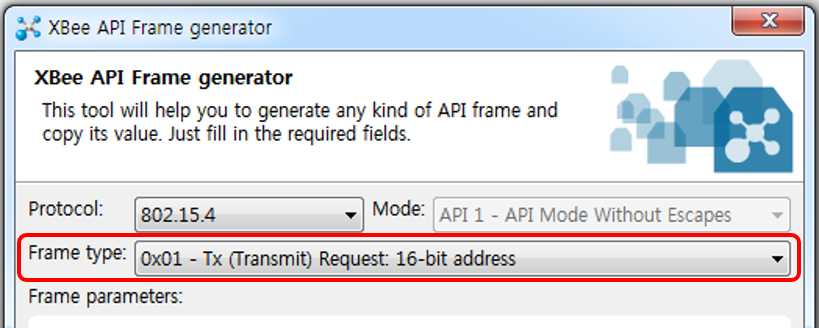
9. Now, you can see a pre-built message in the Generated frame edit control. For your easy understanding of this type of message, refer to the description of TX (Transmit) Request: 16-bit address in the XBee S1 operation manual.
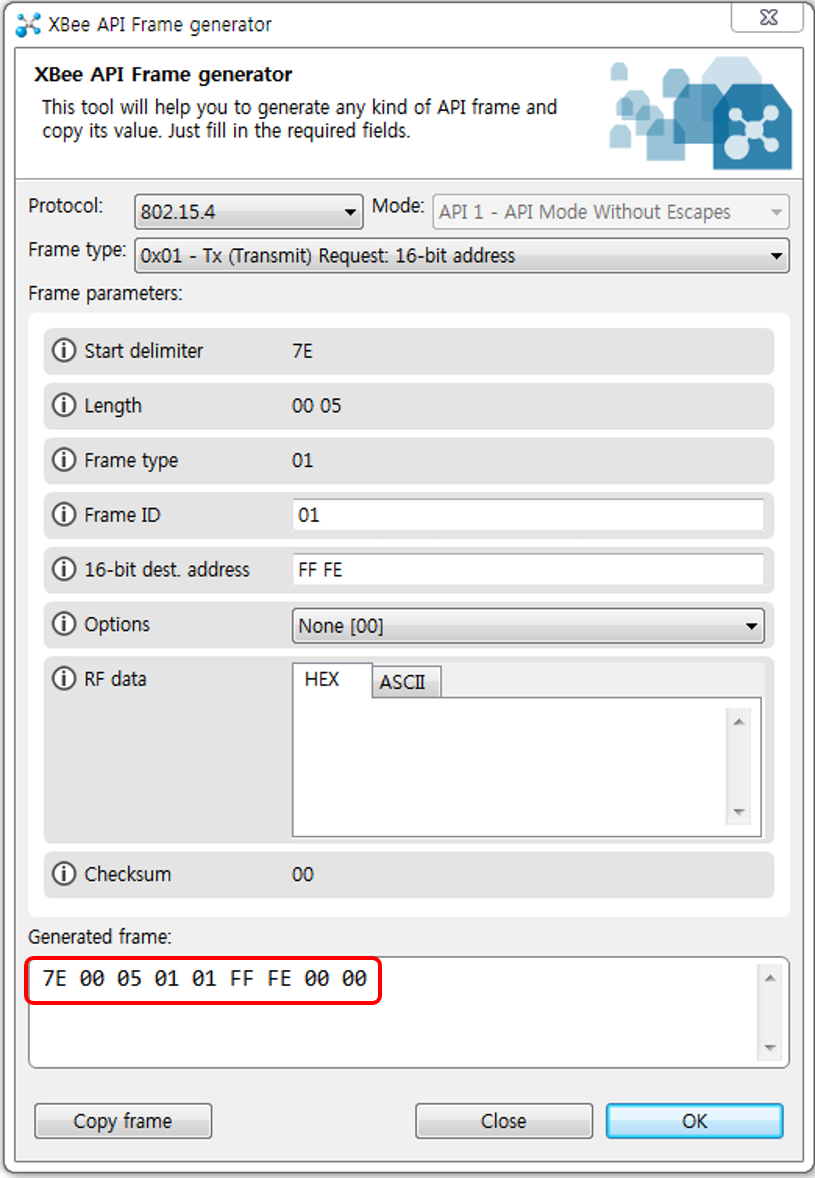
Enter arbitrary Frame ID. We use 56 here. Refer to the XBee S1 operation manual for the meaning of this value.
Enter 16-bit dest. address (destination address, i.e. 16-bit receiver address). Enter 4321 because we will assign 4321 to the receiver as its 16-bit address.
You can add up to 100 bytes of data to be transmitted in the RF data edit control in hexadecimal value or ASCII value. Let's assume that we want to transmit two bytes of data, 0x41 and 0x31. Click HEX tab in RF data edit control and enter 41 and 31. Do not type in the prefix 0x. The value you enter must be hexadecimal numbers.
You can find the updated frame data in the Generated frame edit control.
Click Copy frame button and click OK button.
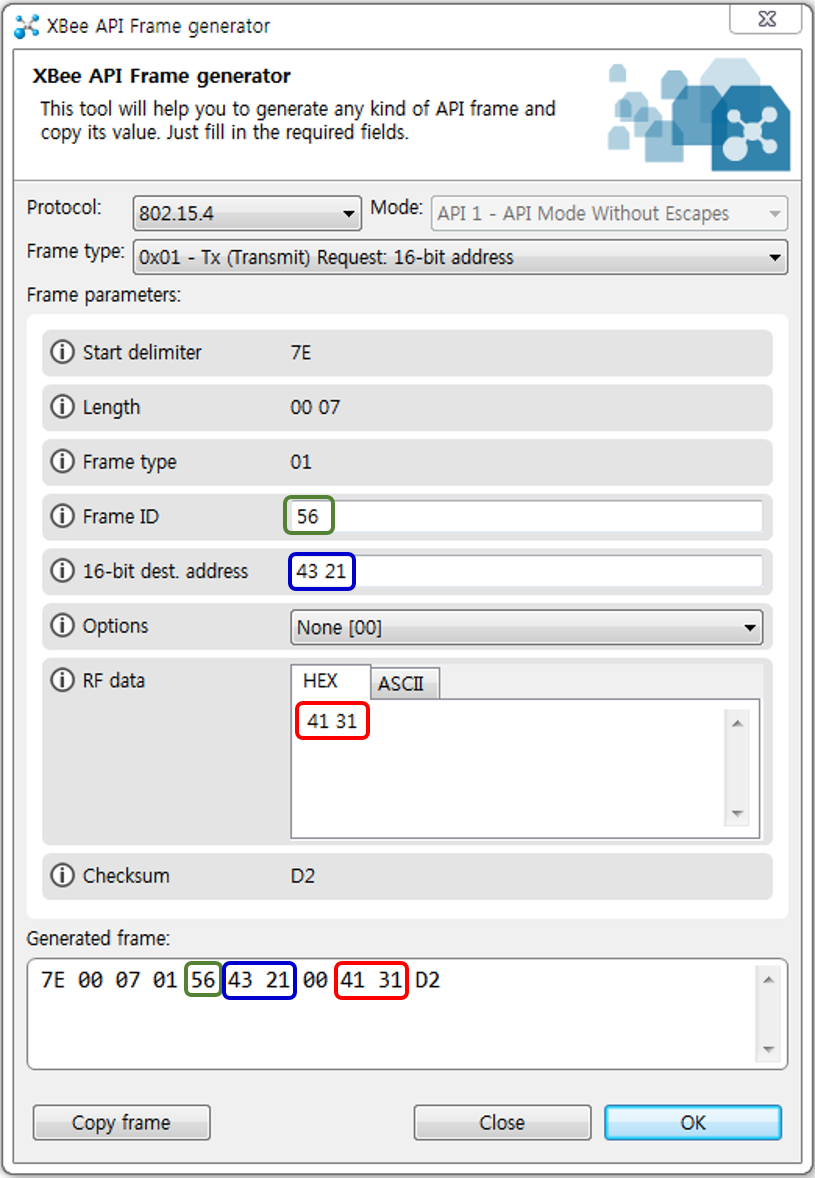
10. Add API frame to the list dialog box is popped up again with the new message frame data. Click Add frame button.
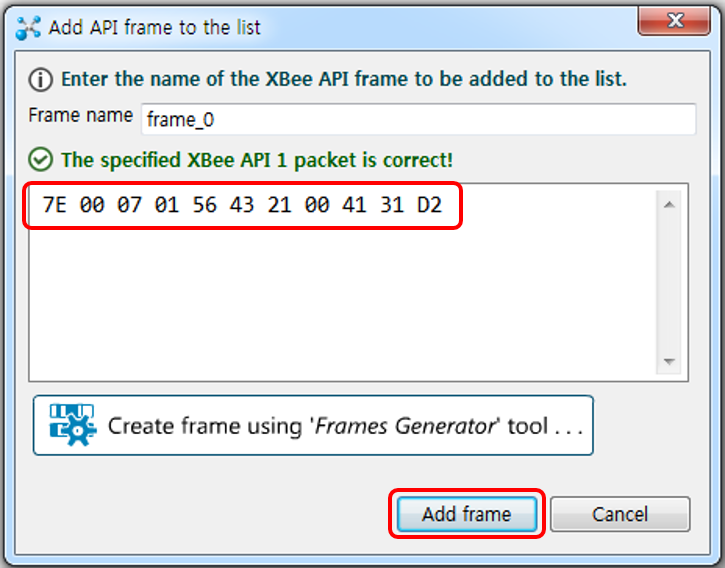
11. Finally, we came back to the main window after composing a new message frame and it is listed in the Send frame list box. Now, we have two options for transmission of this message frame:
A. Send a single frame: a single frame will be transmitted whenever you click Send selected frame button.
B. Send sequence: the selected frame will be transmitted periodically with the time interval specified in the Transmit interval (ms) once you click Start sequence button.
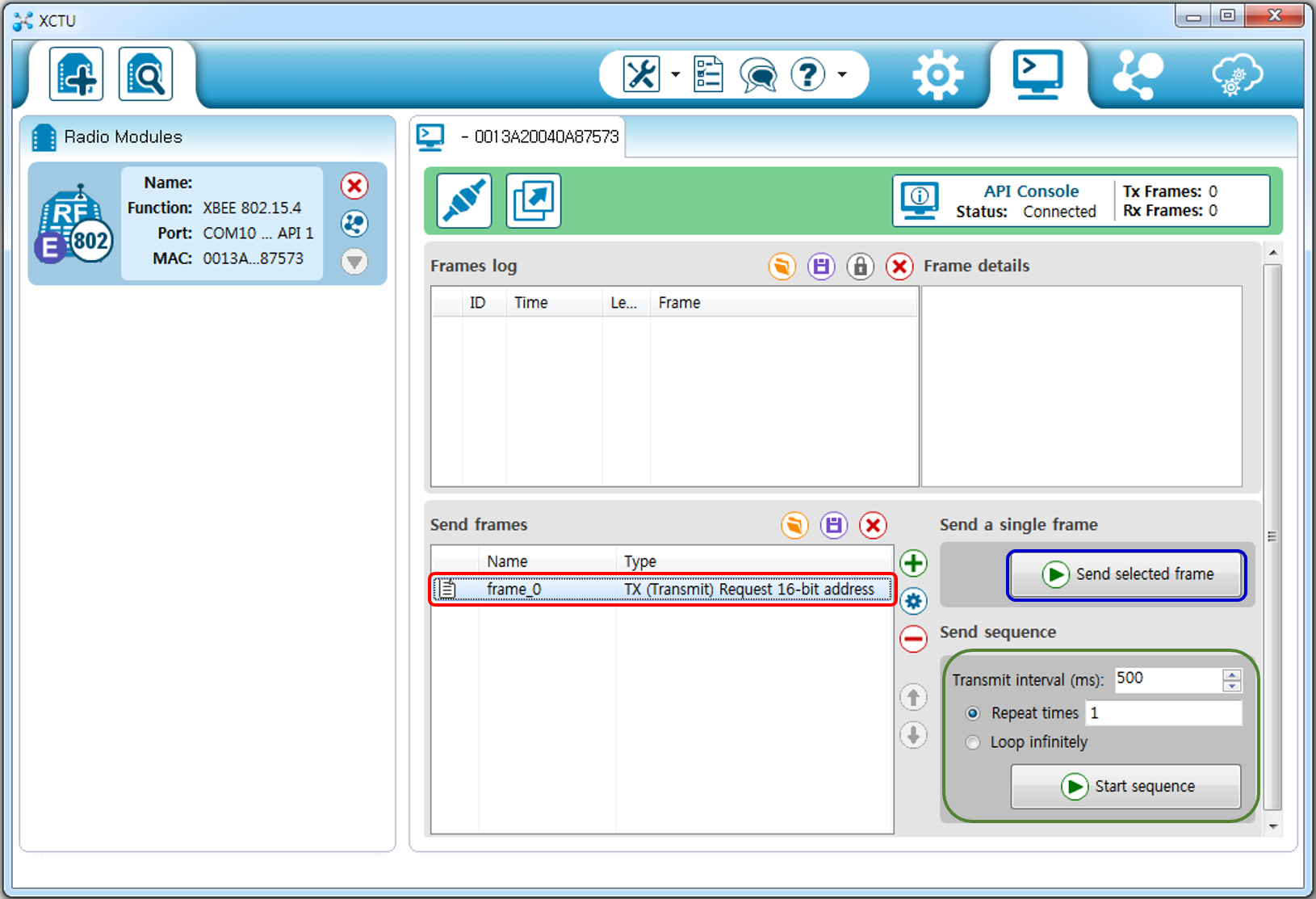
Are you ready to click Send selected frame button and see the received frame data at the receiver? However, we do not have a receiver yet! Let's make a simple receiver to test this transmitter.
12. Let's start with another XBee S1 module which is connected to Arduino's UART port as shown below.
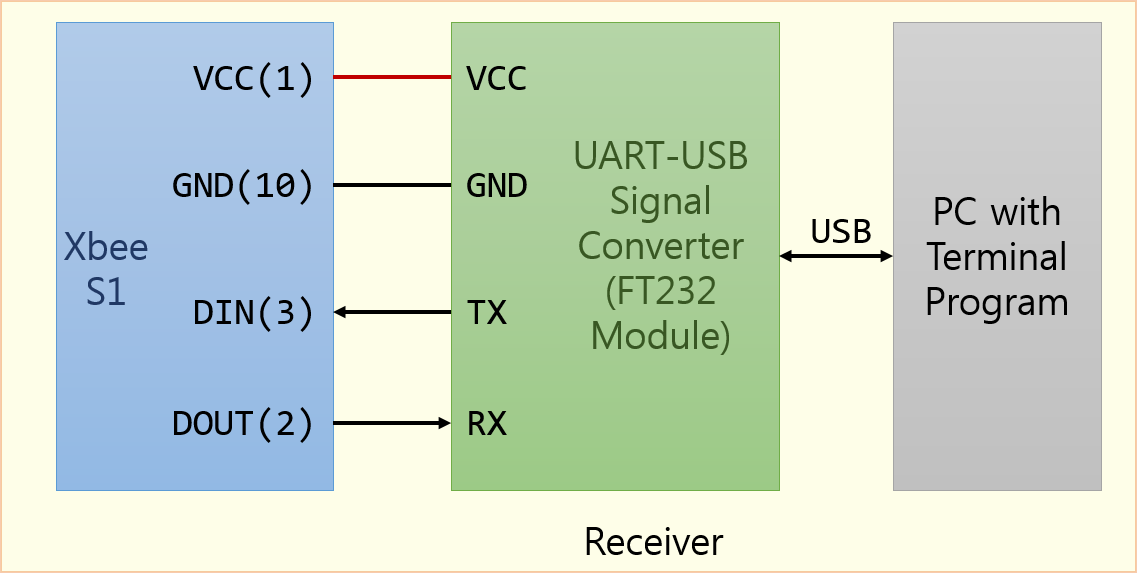
13. The function of the receiver is very simple - it displays all of the received byte data in hexadecimal on the terminal screen of the PC as soon as it receives.
The source code for the receiver is listed below.
Source Code for the Simple Receiver.
/***************************************************** Project : Simple Receiver Version : 0.1 Date : 3/4/2015 Author : JM Cho Chip type : ATmega328P Program type : Application AVR Core Clock frequency: 2.000000 MHz *****************************************************/ #include <mega328p.h> #include <stdio.h> #include <delay.h> #define RX_BUF_LEN 100 char rx_buf[RX_BUF_LEN]; unsigned int wr_ptr; unsigned int rd_ptr; void main(void) { char ch; // Crystal Oscillator division factor: 1. // fclk will be 16MHz/8 = 2MHz CLKPR=0x80; CLKPR=0x03; // USART initialization // Communication Parameters: 8 Data, 1 Stop, No Parity, Baud Rate: 9600 bps // USART Receiver: On, Transmitter: On UCSR0A=0x00; UCSR0B=0x98; // enable RX interrupt UCSR0C=0x06; UBRR0H=0x00; UBRR0L=0x0C; delay_ms(2000); // Set XBee S1 to AT Command mode printf("+++"); delay_ms(2000); printf("ATMY4321\r"); // 0x4321: 16-bit address of this receiver delay_ms(500); printf("ATDL1234\r"); // 0x1234: 16-bit address of the transmitter delay_ms(500); printf("ATDH0\r"); delay_ms(500); printf("ATAP1\r"); // API mode 1 (without escaped. refer to p.89 of XBee User Manual) delay_ms(500); // exit AT command mode printf("ATCN\r"); delay_ms(1000); printf("\nReceiver is ready...\n"); printf("Start transmission...\n"); wr_ptr = rd_ptr = 0; // initialize read pointer and write pointer to rx_buf #asm("sei") // Global enable interrupts while (1) { if (wr_ptr != rd_ptr) // are there received data? { ch = rx_buf[rd_ptr++]; // retrive a received character rd_ptr %= RX_BUF_LEN; // update read pointer if (ch == 0x7E) printf("\n"); printf("0x%02X ", ch); } } } interrupt [USART_RXC] void usart0_rx_isr(void) { rx_buf[wr_ptr++] = UDR0; // store the received character wr_ptr %= RX_BUF_LEN; // update write pointer to rx_buf }
14. Whenever the Send selected frame button described at the step 12 above is clicked, the receiver shows received data in hexadecimal value.
The screen shot of the simple receiver is shown below. Note that the received frame data is not the same as the transmitted frame data. Refer to the description of RX (Receive) Packet: 16-bit Address of the XBee S1 operation manual.
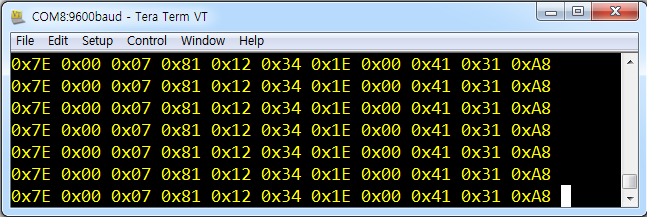
You can find two bytes of data in the received frame, 0x41 and 0x31 in this example, which was sent from the transmitter.
In addition, seventh byte, 0x1E in the screen shot below, indicates RSSI value for each frame. You can use this value for your application.
The fifth and sixth bytes, 0x1234 shows the transmitter's 16-bit address.
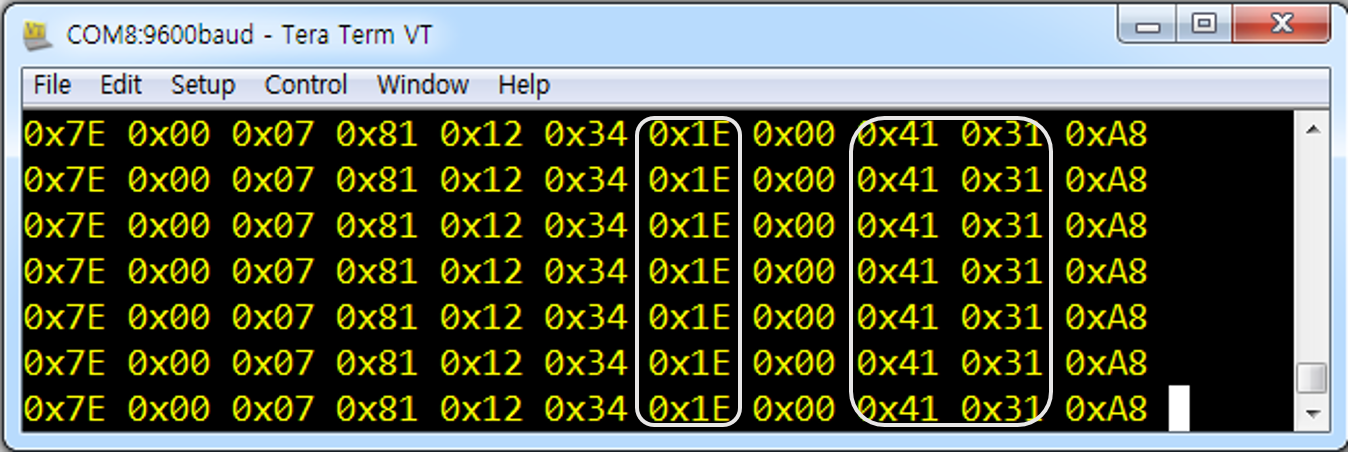
Step II: To Develop an RSSI Measuring Application based on API Mode
In the Step I experiment above we could find that the seventh byte of the received frame indicates the RSSI value when that message frame was received. We will develop an RSSI measuring application based on this value while we are using the same transmitter setup used in the Step I.
1. Modify the source code for the Simple Receiver shown in Step I so that it can extract RSSI value only from the received frame and display it on terminal screen.
Source Code for the RSSI Extractor which will be developed by Adibah comes here.
2. Use the capture function of your terminal program to collect this RSSI data.
3. Repeat Step 2 above for the different position of transmitter XBee S1 module and different distance between the transmitter and receiver.
4. The RSSI value in the collected data changes continuously along the time axis. Create your own algorithms to reduce this noise after analysing the collected data.
5. Integrate all of your experience obtained so far to implement the final receiver that satisfies with your project requirement.
Source Code for your implementation which will be developed by Adibah comes here.
Step III: To Develop an Mobile Transmitter for the RSSI Measuring Application based on API Mode
In the Step I experiment above we set up a transmitter without using a microcontroller and it was stationary.
Sometimes we need a mobile transmitter and its development will be covered in this step.
1. We will use the same message frame created using X-CTU in the Step I. You can create and use your own message frame depending on your application.
2. Set up the Timer1 to generate an interrupt periodically with the time interval required by your application.
3. Whenever Timer1 interrupt occurs, it transmits predefined message frame created in Step 1 above.
4. The ATmega328P and XBee S1 should go to sleep mode when it does not transmit a message frame to save battery power.
Source Code for the mobile transmitter which will be developed by Adibah comes here.
Congratulations!
You can create unlimited number of applications depending on your imagination.
Waiting for your masterpiece...